
CUSTOM POPUPS
RECURSION RE-VISITED
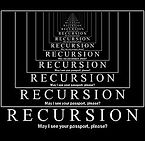
While working, I came across so many road-blocks, turn-a rounds, intelligent code snippets, etc. There can be n number of solutions to a problem, but our intelligence lies in adopting the best and simple one to our scenario. In that process of evaluating, I learnt hell lot of new things. I will try to put forth some of them. In this page itself, you can see that the images are bent a wee bit at the bottom, is it not a neat thing to adopt ??
Learned so far...
We all studied in our academics about Recursion, but then it is a way of making a function or a code snippet calling itself for n number of times (until it achieves the objective) or it reaches the threshold level.
Here we need to understand a few things, 1. What is our objective 2. How to make the function to call itself 3. How to exit from the recursive loop. Also, we have to keep in mind that Recursion doesn't always return success. I will try to explain the same using one of the games that we developed (FlipFlip).
Game:
We have a 8X8 matrix and we have random balls in 5 colors here and there. We need to arrange the balls of the same color in sequence of 4s (or more) either vertically or horizontally. And if we do that, the balls vanish and we make score out of it. We can move only one ball at a time and the ball traverses to a new selected position only if there is a path. So the main logic of this game lies in finding if the path exists b/w the initial and newly selected positions of the ball.
Objective (to find the path (if exists) b/w two positions:
We wrote a function called TRACE that accepts two parameters X and Y (the actual x and y positions of the ball in the matrix).
Exit Condition:
We have to exit the recursion either if the path is traced (success) or we are out of a new position to travel (failure)
Scenario:
We have the ball at 2,2 (second row second column) and the newly selected position is 4,6 and the path exists is (2,2) - (2,3) - (2,4) - (2,5) - (2,6) - (3,6) - (4,6). And from (2,2), the positions (2,1), (2,3), (1,2) and (3,2) are all vacant, so we can go to any of them. The other paths are:
(2,2) - (2,1) - (2,0) - X
(2,2) - (1,2) - X
(2,2) - (3,2) - X Now we will consider the first path where the path stops after (2,0).
Logic::
TRACE(int X, int Y) {
Push the X, Y position into Path Stack
If (X == destinationX && Y == destinationY or Path
Stack is empty)
Return;
Else If (position at X-1 && Y is vacant and if already not visited)
TRACE(X-1, Y);
Else If (position at X+1 && Y is vacant and if already not visited)
TRACE(X-1, Y);
Else If (position at X && Y-1 is vacant and if already not visited)
TRACE(X-1, Y)
Else If (position at X-1 && Y is vacant and if already not visited)
TRACE(X, Y+1);
Else
Pop the position out from the Path Stack and Call TRACE with Top position of the Path Stack
}
** Before calling TRACE initially, push the origin X and Y position into the Path Stack.
Now we push the position (2,2) into the Path Stack and then we call TRACE(2, 2). According to the logic it considers going to (2,1) so TRACE is again called with 2, 1 as parameters.
TRACE(2,2) Path Stack: (2,2)
TRACE(2,1) Push, Path Stack: (2,2) (2,1)
TRACE(2,0) Push, Path Stack: (2,2) (2,1) (2,0)
Pop, Path Stack: (2,2) (2,1)
Pop, Path Stack: (2,2)
TRACE(2,2) Push, Path Stack:(2,2) (2,3)
*
*
*
(2,2) - (2,3) - (2,4) - (2,5) - (2,6) - (3,6) - (4,6)
** We can refine the same algorithm to find the shortest path.
...
...

AND-ENGINE